In this blog post, we’ll explore using the PowerShell ForEach loop and how it can be used to process and manipulate data efficiently.
Understanding the For-Each Loop
The ForEach
loop in PowerShell allows us to iterate over collections of objects, such as arrays, lists, or any other data structure that holds multiple elements. It provides a way to perform a specific set of actions for each item in the collection, making it a fundamental tool for tasks like data processing, automation, and reporting.
Here’s the basic structure of a ForEach
loop in PowerShell:
foreach ($item in $collection) {
#do something with $item
}
Examples Using PowerShell and ForEach
Let’s assume we have a basket of fruit, and we store each piece of fruit in an array of strings. We can then iterate through each fruit like so:
$fruits = @("apple", "banana", "cherry", "peach")
foreach ($fruit in $fruits) {
write-host $fruit
}
In the real-world we might want to loop through files in a directory. In this example we can retrieve all files in a directory using Get-ChildItem
, and then use a ForEach
loop to iterate through the list and write the file path to the console:
$files = Get-ChildItem -Path C:\Alkane\Files
foreach ($file in $files) {
write-host $file.FullName
}
Mastering the ForEach
loop in PowerShell is a crucial skill for anyone looking to automate tasks, process data, or manage resources efficiently.
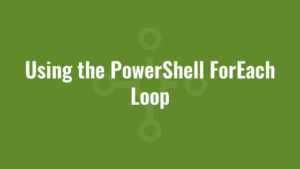
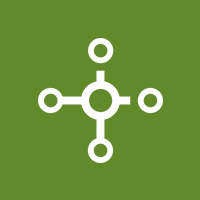