This post explains how we can use PowerShell to restore deleted computer objects from the Active Directory recycle bin.
I usually try to manipulate Active Directory using ADSI, because it doesn’t rely on the Active Directory PowerShell cmdlets. However in this instance time wasn’t on my side, so I installed Remote Server Administration Tools by opening a PowerShell console as my administrator account and running:
Add-WindowsCapability -Name Rsat.ActiveDirectory.DS-LDS.Tools~~~~0.0.1.0 -Online
I then quickly wrote the following script, which takes an array of machines to restore from the Active Directory recycle bin:
$computersToRestore=@("Computer1",
"Computer2",
"Computer3")
cls
foreach($computerToRestore in $computersToRestore) {
write-host "Finding $computerToRestore"
#find the most recent in deleted objects, hence we sort by WhenChanged descending and select the first one
$computer = Get-ADObject -LDAPFilter "(&(objectClass=Computer)(msDS-LastKnownRDN=$computerToRestore*))" -IncludeDeletedObjects -Properties *| Sort-Object -Property WhenChanged -Descending | Select-Object -First 1
if ($computer -ne $null) {
if ($computer.IsDeleted) {
#if we've found the computer in the recycle bin
write-host "Found (in recycle bin): $computerToRestore"
write-host "When Deleted?:" $computer.WhenChanged
write-host "Restoring: $computerToRestore"
Restore-ADObject -Identity $computer
} else {
#this implies the computer has been found, but not in the recycle bin. Hence may already have been restored or was never deleted.
write-host "Found (NOT in recycle bin): $computerToRestore"
}
} else {
#couldn't find computer in AD or in AD recycle bin
write-host "NOT Found $computerToRestore"
}
write-host "***"
}
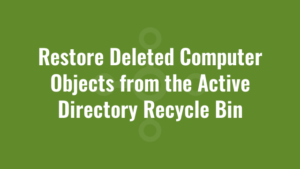
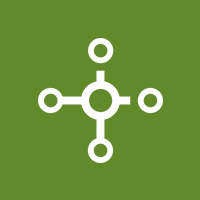